Prompt with AI
Prompt engineering
Prompt engineering involves the design and creation of prompts that are used to elicit specific responses or actions from AI models or interactive systems. These prompts are carefully crafted to guide the behavior or generate particular outputs from the AI, such as generating natural language responses, providing recommendations, or completing specific tasks.
Definition
In the context of AI language models, prompt engineering is especially important for shaping the model's behavior and output. By designing prompts effectively, engineers can influence the model's responses and ensure that it generates coherent, relevant, and accurate content.
There are four main areas to consider when writing an effective prompt. You don’t need to use all four, but using a few will help!
Persona
: Who is the user you're writing for? What are their skills and knowledge?Task
: What specific action do you want the user to perform?Context
: What information does the user need to know to complete the task?Format
: What is the desired output of the task?
Example Prompt:
- [
Persona
] You are a Google Cloud program manager. - [
Task
] Draft an executive summary email - [
Context
] to [person description] based on [details about relevant program docs]. - [
Format
] Limit to bullet points.
By using "act as," you are establishing a specific context for the language model and guiding it to understand the type of task or request you are making. This helps to set the right expectations and provides the language model with the necessary context to generate a response tailored to the defined role.
"Act as a creative writing assistant and generate a short story based
on a prompt about a futuristic world where robots have become sentient."
Framework : Chain of Thought
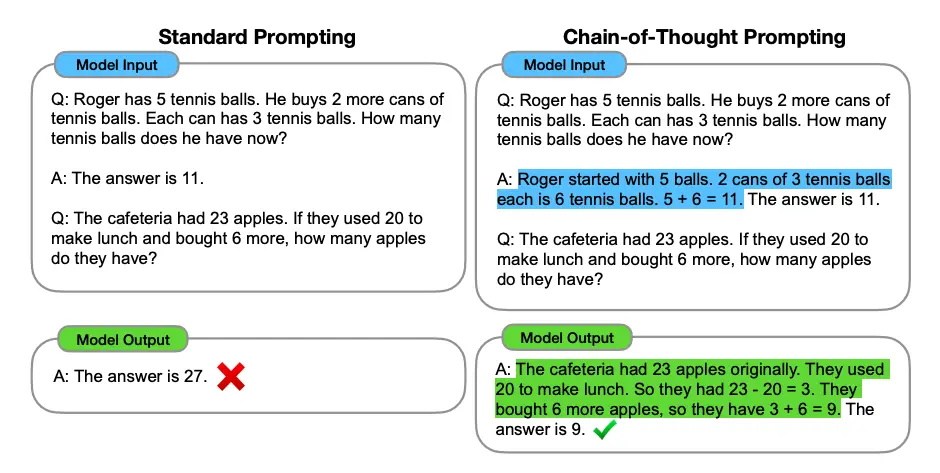
Introduced in Wei et al. (2022), chain-of-thought (CoT) prompting enables complex reasoning capabilities through intermediate reasoning steps. You can combine it with few-shot prompting to get better results on more complex tasks that require reasoning before responding. Prompting Guide with CoT
Framework : ReAct Prompting
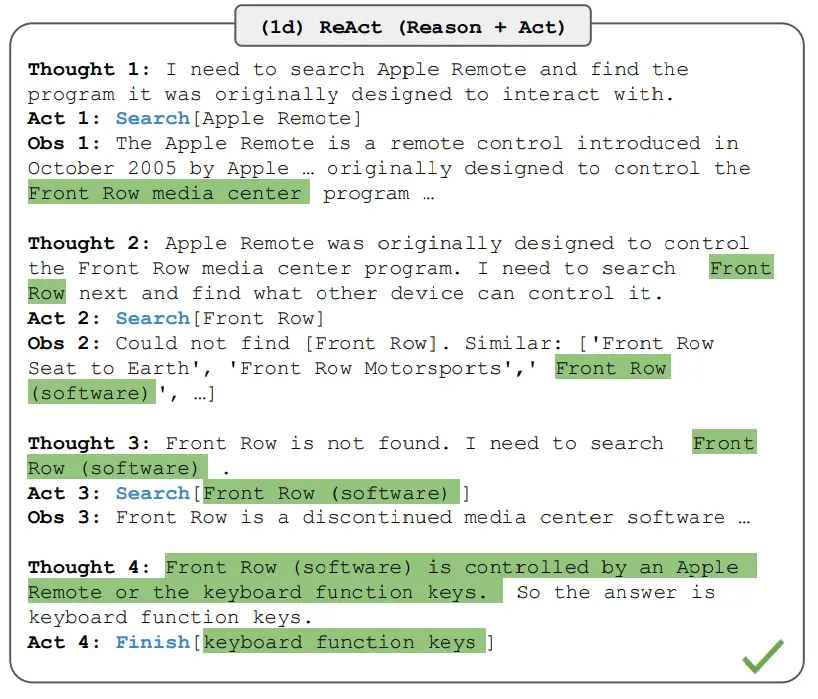
Yao et al., 2022 introduced a framework named ReAct where LLMs are used to generate both reasoning traces and task-specific actions in an interleaved manner.
Generating reasoning traces allow the model to induce, track, and update action plans, and even handle exceptions. The action step allows to interface with and gather information from external sources such as knowledge bases or environments.
The ReAct framework can allow LLMs to interact with external tools to retrieve additional information that leads to more reliable and factual responses. Prompting Guide with CoT
Summarize
Summary is a prompt engineering technique that involves providing a summary of a given document or text. It can helps for summarizing changelogs, articles, or other technical documents.
Help me write an article of this document [Insert or copy paste document text]
Generate 5 titles out of the following topic….
Generate a subtitle to catch readers’ attention on the following
topic [describe the topic]
Write
Write is a prompt engineering technique that involves providing a step-by-step guide or instructions for a given task or process. Its useful for developers to create functional and technical documentations.
Create a template of an email response to customer inquiring about ….
Create a guide that explains how to use ….
Write step by step instructions
For Developers
Code explanation
Code explanation is a prompt engineering technique that involves providing a detailed explanation of a code snippet or function. This technique is useful for developers who want to understand the inner workings of a codebase or for those who want to document their code.
cf. Preformatted prompts for an example of code explanation
Generation
Act as a code generator. Generate a Python function that takes a list of
numbers as input and returns the sum of all the numbers.
🧪 Exercises
Basic Function Creation
- Create a function that calculates the factorial of a number.
- Handle both positive integers and zero, with error handling for negative inputs.
Expected Output (python)
def factorial(n):
if n < 0:
raise ValueError("Input must be a non-negative integer.")
if n == 0:
return 1
result = 1
for i in range(1, n + 1):
result *= i
return result
API Request Handling
- Write a function to fetch data from a given URL using the Fetch API.
- Return the JSON response and handle any potential errors.
Expected Output (javascript)
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error("Error fetching data:", error);
}
}
Class Definition
- Define a class representing a book with properties for title, author, and publication year.
- Include a method to display the book's details.
Expected Output (C#)
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public int PublicationYear { get; set; }
public void DisplayDetails()
{
Console.WriteLine($"Title: {Title}, Author: {Author}, Year: {PublicationYear}");
}
}
Simple Web Server
- Set up a basic web server that listens on port 3000.
- Respond with a simple message when accessed.
Expected Output (Javascript)
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Data Validation
Details:
- Write a method to validate if a given string is a valid email address.
- Use a regular expression for the validation.
Expected Output (Ruby)
def valid_email?(email)
regex = /\A[\w+\-.]+@[a-z\d\-.]+\.[a-z]+\z/i
!!(email =~ regex)
end
Completion
Code completion is a prompt engineering technique that involves providing a list of possible completions for a given code snippet or function. This technique is useful for developers who want to suggest possible code changes or improvements based on their existing code.
Conversion
Code conversion is a prompt engineering technique that involves providing a conversion of a code snippet or function from one programming language to another. This technique is useful for developers who want to migrate their code from one language to another or for those who want to use a different programming language for their projects.
Review
Code review is a prompt engineering technique that involves providing a code review of a given code snippet or function. This technique is useful for developers who want to review their code for potential issues,bugs, or for those who want to provide feedback on their code.
Fixing
Code fixing is a prompt engineering technique that involves providing a code fix for a given code snippet or function. This technique is useful for developers who want to fix bugs or issues in their code or for those who want to improve the quality of their code.
Help me find mistakes in my code [insert your code]
Explain what this snippet of code does [insert code snippet]
What it the correct syntax for a [statement or function]
in [programming language]
How do I fix the following programming language code
[program language] code which explain the functioning [Insert code snippet]
Refactoring
Code refactor is a prompt engineering technique that involves providing a code refactoring of a given code snippet or function within a specific scope. This technique is useful for developers who want to refactor their code within a specific context or for those who want to improve the readability and maintainability of their code.
Data mocking
Mock data generation is a prompt engineering technique that involves providing a mock data set for a given code snippet or function. This technique is useful for developers who want to test their code with mock data or for those who want to generate test data for their projects. It avoid creating manually fake data for testing.
🧪 Exercises
Mock Data Generation
Create prompts that can generate mock user profiles. The language used is JavaScript.
The profile should include:
- Name
- Age
- Address (Street, City, State, Zip Code)
- Phone Number
Go further
Testing
Testing is a prompt engineering technique that involves providing a test case for a given code snippet or function. This technique is useful for developers who want to test their code or for those who want to ensure the correctness of their code.
System design and architecture
System design and architecture is a prompt engineering technique that involves providing a system design or architecture for a given code snippet or function. This technique is useful for developers who want to design their code or for those who want to understand the overall architecture of their projects.
Documentation generation
Documentation generation is a prompt engineering technique that involves providing a documentation for a given code snippet or function. This technique is useful for developers who want to document their code or for those who want to provide documentation for their projects. It can be used to generate documentation in various formats such as Markdown, HTML, or PDF.
Commit Message Generation
Commit message generation is a prompt engineering technique that involves providing a commit message for a given code snippet or function. This technique is useful for developers who want to generate commit messages for their code or for those who want to ensure that their commit messages are clear and concise.
Vulnerability checking
Vulnerability checking is a prompt engineering technique that involves providing a vulnerability check for a given code snippet or function. This technique is useful for developers who want to check for vulnerabilities in their code or for those who want to ensure that their code is secure.
Warning
This prompt is not recommended for production use. It is intended for testing and debugging purposes only and is not a proof of security or safety of your app.
Shell/CLI commands manual
As a developer, you’re not limited to only writing code. LLMs can assist with CLI such as shell commands and version control using Git.
Regular expression explanation
You can understand complex regular expressions and generate ones that match specific patterns in text. This technique is useful for developers who want to write complex regular expressions or for those who want to understand the syntax of regular expressions.
Explain this regular expression in JavaScript: const regex =
/^[A-Za-z0–9._%+-]+@[A-Za-z0–9.-]+\\.[A-Za-z]{2,}$/;